Whatever development method you apply, tests are the only way to ensure that the delivered product conforms to the client’s requirements.
1. Unit Tests
They are the simplest. But hence it is needed to …
-
forget 'manual' approaches
-
explore their 'limitations'
-
treate both the 'qualitative' and 'quantitative' aspects
Let’s consider the implementation of an abstract type: MatrixInt
-
Operations
-
createMatrix : Int * Int → MatrixInt
-
getNbLines : MatrixInt → Int
-
getNbColumns : MatrixInt → Int
-
…
-
-
Preconditions
-
createMatrix(l,c) valid IF and ONLY IF ( l > 0 ) AND ( c > 0 )
-
getElement(m,i,j) valid IF and ONLY IF ( 0 < = i < getNbLines(m) ) AND ( 0 < = j < getNbColumns(m))
-
…
-
-
Axioms
-
getNbLines(createMatrix(l,c)) = l
-
getNbColumns(createMatrix(l,c)) = c
-
getElement(createMatrix(l,c),i,j) = 0
-
isSquared(createMatrix(l,c)) IF and ONLY IF l = c
-
…
-
Spécifications
Opérations
Preconditions
|
import junit.textui.TestRunner;
import junit.framework.TestSuite;
import junit.framework.TestCase;
public class MatriceEntierOperationsTest extends TestCase {
static int totalAssertions = 0;
static int bilanAssertions = 0;
/*
Types des opérations du type MatriceEntier
*/
public void test_type_new_MatriceEntier() throws Exception {
MatriceEntier m = new MatriceEntier(3,3) ;
totalAssertions++ ;
assertEquals("new MatriceEntier(3,3) retourne une MatriceEntier", "MatriceEntier", m.getClass().getName());
bilanAssertions++ ;
}
public void test_type_get() throws Exception {
MatriceEntier m = new MatriceEntier(3,4) ;
totalAssertions++ ;
assertTrue("getNbLignes() > 0", m.getNbLignes() > 0);
bilanAssertions++ ;
totalAssertions++ ;
assertTrue("getNbColonnes() > 0", m.getNbColonnes() > 0);
bilanAssertions++ ;
for (int i=0; i<m.getNbLignes(); i++) {
for (int j=0; j<m.getNbColonnes(); j++) {
totalAssertions++ ;
assertTrue("getElement() retourne un entier", (m.getElement(i,j) >= 0) || (m.getElement(i,j) < 0));
bilanAssertions++ ;
}
}
}
public void test_type_som() throws Exception {
MatriceEntier m = new MatriceEntier(3,4) ;
for (int i=0; i<m.getNbLignes(); i++) {
totalAssertions++ ;
assertTrue("somLigne("+i+") >= 0", m.somLigne(i) >= 0);
bilanAssertions++ ;
}
for (int j=0; j<m.getNbColonnes(); j++) {
totalAssertions++ ;
assertTrue("somColonne("+j+") >= 0", m.somColonne(j) >= 0);
bilanAssertions++ ;
}
}
public void test_type_est() throws Exception {
MatriceEntier m = new MatriceEntier(3,3) ;
totalAssertions++ ;
assertTrue("estCarree() retourne un booleen", (m.estCarree() == true) || (m.estCarree() == false));
bilanAssertions++ ;
totalAssertions++ ;
assertTrue("estDiagonale() retourne un booleen", (m.estDiagonale() == true) || (m.estDiagonale() == false));
bilanAssertions++ ;
m.setElement(0,0,1) ;
totalAssertions++ ;
assertTrue("estDiagonale() retourne un booleen", (m.estDiagonale() == true) || (m.estDiagonale() == false));
bilanAssertions++ ;
m = new MatriceEntier(3,4) ;
totalAssertions++ ;
assertTrue("estCarree() retourne un booleen", (m.estCarree() == true) || (m.estCarree() == false));
bilanAssertions++ ;
}
public void test_type_set_mul() throws Exception {
MatriceEntier m = new MatriceEntier(3,3) ;
for (int i=0; i<m.getNbLignes(); i++) {
for (int j=0; j<m.getNbColonnes(); j++) {
totalAssertions++ ;
assertEquals("setElement() retourne une MatriceEntier", "MatriceEntier", m.setElement(i,j,i+j).getClass().getName());
bilanAssertions++ ;
}
}
totalAssertions++ ;
assertEquals("setPremiereDiagonale(99) retourne une MatriceEntier", "MatriceEntier", m.setPremiereDiagonale(99).getClass().getName());
bilanAssertions++ ;
totalAssertions++ ;
assertEquals("setSecondeDiagonale(99) retourne une MatriceEntier", "MatriceEntier", m.setSecondeDiagonale(99).getClass().getName());
bilanAssertions++ ;
totalAssertions++ ;
assertEquals("mulMatNombre() retourne une MatriceEntier", "MatriceEntier", m.mulMatNombre(33).getClass().getName());
bilanAssertions++ ;
}
/*
main() de la classe de Test
*/
public static void main(String[] args) {
junit.textui.TestRunner.run(new TestSuite(MatriceEntierOperationsTest.class));
if (bilanAssertions == totalAssertions) { System.out.print("Bravo !"); }
else { System.out.print("OUPS !"); }
System.out.println(" "+bilanAssertions+"/"+totalAssertions+" assertions verifiees");
} // fin main
} // fin MatriceEntierOperationsTest
import junit.textui.TestRunner;
import junit.framework.TestSuite;
import junit.framework.TestCase;
public class MatriceEntierPreconditionsTest extends TestCase {
static int totalAssertions = 0;
static int bilanAssertions = 0;
/*
Préconditions du type Pile
*/
public void test_precondition1() {
MatriceEntier m ;
boolean exception = false ;
try { m = new MatriceEntier(0,1) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("new MatriceEntier(0,1) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m = new MatriceEntier(1,0) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("new MatriceEntier(1,0) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m = new MatriceEntier(0,0) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("new MatriceEntier(0,0) leve une exception", exception);
bilanAssertions++ ;
}
public void test_precondition2() throws Exception {
MatriceEntier m = new MatriceEntier(2,3);
boolean exception = false ;
try { m.getElement(-1,1) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("getElement(-1,1) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.getElement(2,2) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("getElement(2,2) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.getElement(1,-1) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("getElement(1,-1) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.getElement(1,3) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("getElement(1,3) leve une exception", exception);
bilanAssertions++ ;
}
public void test_precondition3() throws Exception {
MatriceEntier m = new MatriceEntier(2,3);
boolean exception = false ;
try { m.setElement(-1,1,99) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("setElement(-1,1,99) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.setElement(2,2,99) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("setElement(2,2,99) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.setElement(1,-1,99) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("setElement(1,-1,99) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.setElement(1,3,99) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("setElement(1,3,99) leve une exception", exception);
bilanAssertions++ ;
}
public void test_precondition4() throws Exception {
MatriceEntier m = new MatriceEntier(2,3);
boolean exception = false ;
try { m.somLigne(-1) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("somLigne(-1) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.somLigne(2) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("somLigne(2) leve une exception", exception);
bilanAssertions++ ;
}
public void test_precondition5() throws Exception {
MatriceEntier m = new MatriceEntier(2,3);
boolean exception = false ;
try { m.somColonne(-1) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("somColonne(-1) leve une exception", exception);
bilanAssertions++ ;
exception = false ;
try { m.somColonne(3) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("somColonne(3) leve une exception", exception);
bilanAssertions++ ;
}
public void test_precondition6() throws Exception {
MatriceEntier m = new MatriceEntier(2,3);
boolean exception = false ;
try { m.setPremiereDiagonale(99) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("setPremiereDiagonale(99) leve une exception", exception);
bilanAssertions++ ;
}
public void test_precondition7() throws Exception {
MatriceEntier m = new MatriceEntier(2,3);
boolean exception = false ;
try { m.setSecondeDiagonale(99) ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("setSecondeDiagonale(99) leve une exception", exception);
bilanAssertions++ ;
}
public void test_precondition8() throws Exception {
MatriceEntier m = new MatriceEntier(2,3);
boolean exception = false ;
try { m.estDiagonale() ; }
catch (Exception e) { exception = true ; };
totalAssertions++ ;
assertTrue("estDiagonale() leve une exception", exception);
bilanAssertions++ ;
}
/*
main() de la classe de Test
*/
public static void main(String[] args) {
junit.textui.TestRunner.run(new TestSuite(MatriceEntierPreconditionsTest.class));
if (bilanAssertions == totalAssertions) { System.out.print("Bravo !"); }
else { System.out.print("OUPS !"); }
System.out.println(" "+bilanAssertions+"/"+totalAssertions+" assertions verifiees");
} // fin main
} // fin MatriceEntierPreconditionsTest
import junit.textui.TestRunner;
import junit.framework.TestSuite;
import junit.framework.TestCase;
public class MatriceEntierAxiomesTest extends TestCase {
static int totalAssertions = 0;
static int bilanAssertions = 0;
/*
Axiomes du type MatriceEntier
*/
public void test_get() throws Exception {
MatriceEntier m = new MatriceEntier(3,4) ;
totalAssertions++ ;
assertEquals("getNbLignes() == 3", 3, m.getNbLignes());
bilanAssertions++ ;
totalAssertions++ ;
assertEquals("getNbColonnes() == 4", 4, m.getNbColonnes());
bilanAssertions++ ;
for (int i=0; i<m.getNbLignes(); i++) {
for (int j=0; j<m.getNbColonnes(); j++) {
totalAssertions++ ;
assertEquals("getElement("+i+","+j+") == 0", 0, m.getElement(i,j));
bilanAssertions++ ;
}
}
m = new MatriceEntier(3,3) ;
totalAssertions++ ;
assertEquals("setPremiereDiagonale(99).getNbLignes() == getNbLignes()", m.setPremiereDiagonale(99).getNbLignes(), m.getNbLignes());
bilanAssertions++ ;
totalAssertions++ ;
assertEquals("setSecondeDiagonale(99).getNbLignes() == getNbLignes()", m.setSecondeDiagonale(99).getNbLignes(), m.getNbLignes());
bilanAssertions++ ;
m = new MatriceEntier(3,3) ;
m.setPremiereDiagonale(99) ;
for (int i=0; i<m.getNbLignes(); i++) {
for (int j=0; j<m.getNbColonnes(); j++) {
totalAssertions++ ;
if ( i == j ) {
assertEquals("getElement("+i+","+i+") == 99", 99, m.getElement(i,i));
} else {
assertEquals("getElement("+i+","+j+") == 0", 0, m.getElement(i,j));
}
bilanAssertions++ ;
}
}
} // fin test_get
public void test_som() throws Exception {
MatriceEntier m = new MatriceEntier(3,4) ;
for (int i=0; i<m.getNbLignes(); i++) {
totalAssertions++ ;
assertEquals("somLigne("+i+") == 0", 0, m.somLigne(i));
bilanAssertions++ ;
}
for (int j=0; j<m.getNbColonnes(); j++) {
totalAssertions++ ;
assertEquals("somColonne("+j+") == 0", 0, m.somColonne(j));
bilanAssertions++ ;
}
m = new MatriceEntier(3,3) ;
m.setPremiereDiagonale(99) ;
for (int ij=0; ij<m.getNbLignes(); ij++) {
totalAssertions = totalAssertions + 2 ; ;
assertEquals("setPremiereDiagonale(99).somLigne("+ij+") == 99", 99, m.somLigne(ij));
bilanAssertions++ ;
assertEquals("setPremiereDiagonale(99).somColonne("+ij+") == 99", 99, m.somColonne(ij));
bilanAssertions++ ;
}
m = new MatriceEntier(3,3) ;
m.setSecondeDiagonale(99) ;
for (int ij=0; ij<m.getNbLignes(); ij++) {
totalAssertions = totalAssertions + 2 ; ;
assertEquals("setSecondeDiagonale(99).somLigne("+ij+") == 99", 99, m.somLigne(ij));
bilanAssertions++ ;
assertEquals("setSecondeDiagonale(99).somColonne("+ij+") == 99", 99, m.somColonne(ij));
bilanAssertions++ ;
}
} // fin test_som
public void test_est() throws Exception {
MatriceEntier m = new MatriceEntier(3,3) ;
totalAssertions++ ;
assertTrue("estCarree() == true", m.estCarree());
bilanAssertions++ ;
m = new MatriceEntier(2,3) ;
totalAssertions++ ;
assertFalse("estCarree() == false", m.estCarree());
bilanAssertions++ ;
m = new MatriceEntier(3,3) ;
totalAssertions++ ;
assertTrue("estDiagonale() == true", m.estDiagonale());
bilanAssertions++ ;
m.setPremiereDiagonale(99);
totalAssertions++ ;
assertTrue("setPremiereDiagonale(99).estDiagonale() == true", m.estDiagonale());
bilanAssertions++ ;
m.setSecondeDiagonale(99);
totalAssertions++ ;
assertFalse("setSecondeDiagonale(99).estDiagonale() == false", m.estDiagonale());
bilanAssertions++ ;
} // fin test_est
public void test_mul() throws Exception {
MatriceEntier m = new MatriceEntier(3,3) ;
m.setPremiereDiagonale(1).mulMatNombre(99) ;
for (int ij=0; ij<m.getNbLignes(); ij++) {
totalAssertions = totalAssertions + 2 ; ;
assertEquals("setPremiereDiagonale(1).mulMatNombre(99).somLigne("+ij+") == 99", 99, m.somLigne(ij));
bilanAssertions++ ;
assertEquals("setPremiereDiagonale(1).mulMatNombre(99).somColonne("+ij+") == 99", 99, m.somColonne(ij));
bilanAssertions++ ;
}
m = new MatriceEntier(3,3) ;
m.setSecondeDiagonale(1).mulMatNombre(99) ;
for (int ij=0; ij<m.getNbLignes(); ij++) {
totalAssertions = totalAssertions + 2 ; ;
assertEquals("setSecondeDiagonale(1).mulMatNombre(99).somLigne("+ij+") == 99", 99, m.somLigne(ij));
bilanAssertions++ ;
assertEquals("setSecondeDiagonale(1).mulMatNombre(99).somColonne("+ij+") == 99", 99, m.somColonne(ij));
bilanAssertions++ ;
}
MatriceEntier m_init = new MatriceEntier(3,3) ;
m = new MatriceEntier(3,3) ;
// Initialise m_init et m à {{0,1,2}{3,4,5}{6,7,8}}
int k = 0 ;
for (int i=0; i<m.getNbLignes(); i++) {
for (int j=0; j<m.getNbColonnes(); j++) {
m_init.setElement(i,j,k) ;
m.setElement(i,j,k) ;
k = k + 1 ;
}
}
m.mulMatNombre(3) ;
for (int i=0; i<m.getNbLignes(); i++) {
for (int j=0; j<m.getNbColonnes(); j++) {
totalAssertions++ ;
assertEquals("m.mulMatNombre(3).getElement("+i+","+j+") == m.getElement("+i+","+j+") * 3", m_init.getElement(i,j) * 3, m.getElement(i,j));
bilanAssertions++ ;
}
}
} // fin test_mul
/*
main() de la classe de Test
*/
public static void main(String[] args) {
junit.textui.TestRunner.run(new TestSuite(MatriceEntierAxiomesTest.class));
if (bilanAssertions == totalAssertions) { System.out.print("Bravo !"); }
else { System.out.print("OUPS !"); }
System.out.println(" "+bilanAssertions+"/"+totalAssertions+" assertions verifiees");
} // fin main
} // fin MatriceEntierAxiomesTest
import junit.textui.TestRunner;
import junit.framework.TestSuite;
import junit.framework.TestCase;
public class MatriceEntierOpSupTest extends TestCase {
static int totalAssertions = 0;
static int bilanAssertions = 0;
/*
Opérations supplémentaires du type MatriceEntier
*/
public void test_toString() throws Exception {
MatriceEntier m = new MatriceEntier(3,3) ;
m.setPremiereDiagonale(1).setSecondeDiagonale(2) ;
String ln = System.getProperty("line.separator") ;
String attendu = "1 0 2 " + ln + "0 2 0 " + ln + "2 0 1 " + ln ;
totalAssertions++ ;
assertEquals("toString() == ", attendu, m.toString());
bilanAssertions++ ;
}
public void test_toHTML() throws Exception {
MatriceEntier m = new MatriceEntier(3,3) ;
m.setPremiereDiagonale(1).setSecondeDiagonale(2) ;
String ln = System.getProperty("line.separator") ;
String attendu = "<table border=\"1\">" + ln ;
attendu += "<tr><td>1</td><td>0</td><td>2</td></tr>" + ln + "<tr><td>0</td><td>2</td><td>0</td></tr>" + ln + "<tr><td>2</td><td>0</td><td>1</td></tr>" + ln ;
attendu += "</table>" + ln ;
totalAssertions++ ;
assertEquals("toHTML() == ", attendu, m.toHTML());
bilanAssertions++ ;
}
/*
main() de la classe de Test
*/
public static void main(String[] args) {
junit.textui.TestRunner.run(new TestSuite(MatriceEntierOpSupTest.class));
if (bilanAssertions == totalAssertions) { System.out.print("Bravo !"); }
else { System.out.print("OUPS !"); }
System.out.println(" "+bilanAssertions+"/"+totalAssertions+" assertions verifiees");
} // fin main
} // fin PileTest
The testing program is :
-
a regression detection tool
-
that should be run on each modification of the
MatrixInt
class
-
-
a specification documentation
-
precise AND concise
-
-
a programmers' documentation
-
operational
-
2. Integration Tests
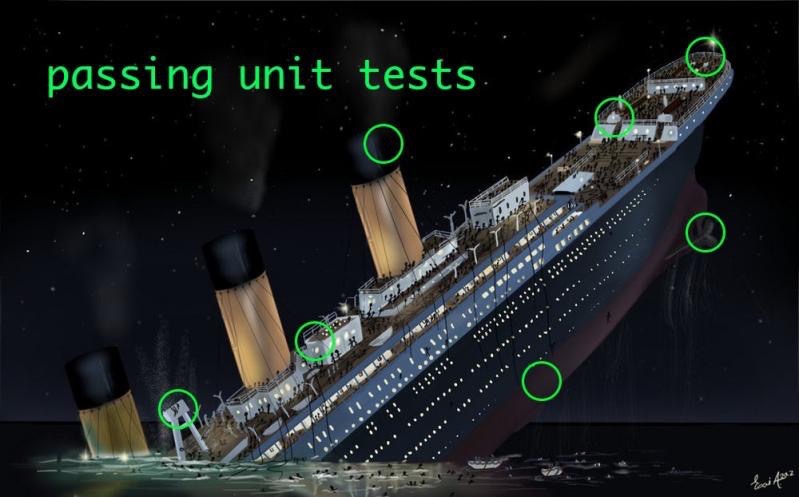
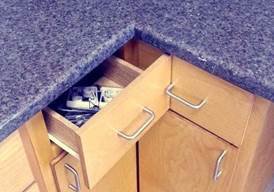
It a more complicated activity. It is required to:
-
test the client’s expectations/requirements
-
test systems' interactions
-
no redo the unit tests
JourSuivantAvecLibDate.class
import junit.textui.TestRunner;
import junit.framework.TestSuite;
import junit.framework.TestCase;
import java.io.*;
public class JourSuivantAvecLibDateTest extends TestCase {
static String programmeATester = "JourSuivantAvecLibDate" ; (1)
Process executionProgrammeATester ; (2)
BufferedReader ecranProgrammeATester ; (3)
BufferedWriter clavierProgrammeATester ; (4)
String finDeLigne = System.getProperty("line.separator") ; (5)
public static void main(String[] args) {
if ( args.length > 0 ) { programmeATester = args[0] ; }
System.out.println("Tests du programme : " + programmeATester);
junit.textui.TestRunner.run(new TestSuite(JourSuivantAvecLibDateTest.class)); (6)
}
protected void setUp() throws IOException { (7)
executionProgrammeATester = Runtime.getRuntime().exec("java -cp . "+programmeATester); (8)
ecranProgrammeATester = new BufferedReader(new InputStreamReader( executionProgrammeATester.getInputStream() )); (9)
clavierProgrammeATester = new BufferedWriter(new OutputStreamWriter( executionProgrammeATester.getOutputStream() )); (10)
}
// Saisies valides
public void test_31_1_2013() throws IOException {
assertEquals("Affiche : 'Saisir une date : jour mois annee ? '","Saisir une date : jour mois annee ? ",ecranProgrammeATester.readLine()); (11)
clavierProgrammeATester.write("31 1 2013"+finDeLigne); (12)
clavierProgrammeATester.flush(); (13)
assertEquals("Affiche : 'Le lendemain du 31/1/2013'","Le lendemain du 31/1/2013",ecranProgrammeATester.readLine());
assertEquals("Affiche : 'sera le 1/2/2013.'","sera le 1/2/2013.",ecranProgrammeATester.readLine()); (14)
}
public void test_28_2_2013() throws IOException {
String messageSaisie = "Saisir une date : jour mois annee ? " ;
String[] ligneJeuDEssai = {"28 2 2013","Le lendemain du 28/2/2013","sera le 1/3/2013."} ;
assertEquals("Affiche : "+messageSaisie,messageSaisie,ecranProgrammeATester.readLine());
clavierProgrammeATester.write(ligneJeuDEssai[0]+finDeLigne); clavierProgrammeATester.flush();
assertEquals("Affiche : "+ligneJeuDEssai[1],ligneJeuDEssai[1],ecranProgrammeATester.readLine());
assertEquals("Affiche : "+ligneJeuDEssai[2],ligneJeuDEssai[2],ecranProgrammeATester.readLine());
}
protected void assertsPourSaisieValide(String messageSaisie,String saisie,String affichage1,String affichage2) throws IOException {
assertEquals("Affiche : "+messageSaisie,messageSaisie,ecranProgrammeATester.readLine());
clavierProgrammeATester.write(saisie+finDeLigne); clavierProgrammeATester.flush();
assertEquals("Affiche : "+affichage1,affichage1,ecranProgrammeATester.readLine());
assertEquals("Affiche : "+affichage2,affichage2,ecranProgrammeATester.readLine());
}
public void test_31_3_2013() throws IOException {
String messageSaisie = "Saisir une date : jour mois annee ? " ;
String[] ligneJeuDEssai = {"31 3 2013","Le lendemain du 31/3/2013","sera le 1/4/2013."} ;
assertsPourSaisieValide(messageSaisie,ligneJeuDEssai[0],ligneJeuDEssai[1],ligneJeuDEssai[2]);
}
// Saisies invalides
protected void assertsPourSaisieInvalide(String messageSaisie,String saisie,String affichage) throws IOException {
assertEquals("Affiche : "+messageSaisie,messageSaisie,ecranProgrammeATester.readLine());
clavierProgrammeATester.write(saisie+finDeLigne); clavierProgrammeATester.flush();
assertEquals("Affiche : "+affichage,affichage,ecranProgrammeATester.readLine());
}
public void test_1_1_1581() throws IOException {
String messageSaisie = "Saisir une date : jour mois annee ? " ;
String[] ligneJeuDEssai = {"1 1 1581","La date du 1/1/1581 n'est pas une date valide."} ;
assertsPourSaisieInvalide(messageSaisie,ligneJeuDEssai[0],ligneJeuDEssai[1]);
}
public void test_32_1_2013() throws IOException {
String messageSaisie = "Saisir une date : jour mois annee ? " ;
String[] ligneJeuDEssai = {"32 1 2013","La date du 32/1/2013 n'est pas une date valide."} ;
assertsPourSaisieInvalide(messageSaisie,ligneJeuDEssai[0],ligneJeuDEssai[1]);
}
} // fin class
1 | name of the app to be tested |
2 | Process = the running program |
3 | link to the display of the running program |
4 | link to the keyboard of the running program |
5 | portable carrier return |
6 | launching of all the functions starting by 'test' |
7 | function (re-)executed before each testing function AND launch the program to be tested |
8 | launching of the program |
9 | connect to the display |
10 | connect to the keyboard |
11 | read a line on the display |
12 | send a line to the keyboard |
13 | force the keyboard flush |
14 | read another line on the display |
3. Tests implement simple algorithms
public void test_dates_invalids() {
int[][] tabJeuDEssaiDatesInvalids = { (1)
{1,1,1581},{0,1,2013},{99,99,2099},
{32,1,2013},{29,2,2013},{32,3,2013},
{31,4,2013},{32,5,2013},{31,6,2013},
{32,7,2013},{32,8,2013},{31,9,2013},
{32,10,2013},{31,11,2013},{32,12,2013},
{29,2,1900},{30,2,2000}
} ;
for ( int indice = 0, taille = tabJeuDEssaiDatesInvalids.length;
indice < taille ;
indice = indice + 1){
int[] date = tabJeuDEssaiDatesInvalids[indice] ;
assertFalse(date[0]+"/"+date[1]+"/"+date[2]+" est invalid"
, LibDate.datevalid(date[0],date[1],date[2])); (2) (3)
}
bilanAssertions = bilanAssertions + tabJeuDEssaiDatesInvalids.length ;
}
1 | given: in the following situations |
2 | when: when we check the validity of a date |
3 | then: we should get false |
4. Can everything be tested ?
-
librairies
-
system interactions (concurrency, etc.)
-
network services
-
graphical interfaces (html, java, flash, etc.)
-
…
-
MAY BE NOT, but try anyway!
5. All the strategies are exploitable
|
6. Most common mistakes in programming
Quizz
QUESTION
|